35 Scenario based .Loc Panda Interview Questions: .loc Function in Pandas Deep dive and interviews questions
Pandas, the popular data manipulation and analysis library in Python, provides a plethora of functions to work with data efficiently. One such essential function is loc
, which allows you to access a group of rows and columns in a DataFrame by labels or a boolean array. This function is incredibly versatile and forms a cornerstone of many data analysis tasks. Let's explore the loc
function in detail with various examples.
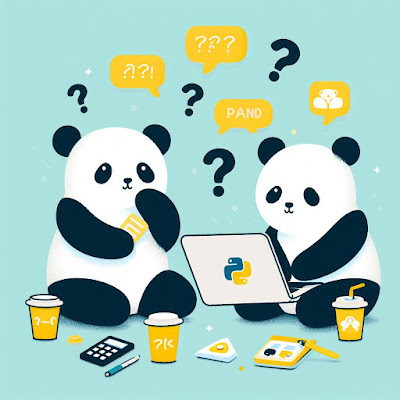
Basic Syntax:
The basic syntax of the loc
function is as follows:
df.loc[row_label, column_label]
Here, df
represents the DataFrame, and row_label
and column_label
can be single labels or lists/arrays of labels.
Examples:
Example 1: Selecting a Single Row
import pandas as pd
# Creating a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'City': ['New York', 'London', 'Paris', 'Tokyo']
}
df = pd.DataFrame(data)
# Using loc to select a single row by label
row_data = df.loc[1]
print(row_data)
In this example, the loc
function is used to select the row with label 1
from the DataFrame df
.
Example 2: Selecting Specific Rows and Columns
# Selecting specific rows and columns using loc
selected_data = df.loc[[0, 2], ['Name', 'City']]
print(selected_data)
Here, the loc
function is used to select rows with labels 0
and 2
and columns 'Name'
and 'City'
.
Example 3: Using Boolean Indexing
# Using boolean indexing with loc
filtered_data = df.loc[df['Age'] > 30, ['Name', 'City']]
print(filtered_data)
In this example, the loc
function is combined with boolean indexing to select rows where the 'Age'
column is greater than 30
, and columns 'Name'
and 'City'
are retrieved.
Example 4: Adding a New Column:
# Adding a new column to the DataFrame
df['Salary'] = [50000, 55000, 60000, 65000]
print("DataFrame with Salary Column:")
print(df)
In this example, a new column named 'Salary'
is added to the DataFrame df
.
Example 5: Removing a Column:
# Removing the 'Age' column from the DataFrame
df.drop('Age', axis=1, inplace=True)
print("DataFrame after removing the 'Age' column:")
print(df)
The drop
function is used to remove the 'Age'
column from the DataFrame df
.
Example 6: Setting a New Index:
# Setting a new index for the DataFrame
new_index = ['A', 'B', 'C', 'D']
df.set_index(pd.Index(new_index), inplace=True)
print("DataFrame with New Index:")
print(df)
Exploring Advanced Data Manipulation with Pandas' .loc
Function
When it comes to sophisticated data manipulation in Python, the .loc
function in the pandas library stands out as a versatile and indispensable tool. Let's dive into some advanced scenarios where the .loc
function shines, empowering data scientists and analysts to handle complex data operations with ease.
Scenario 1: Conditional Data Selection
Filtering data based on specific conditions is a common requirement. With .loc
, you can do this efficiently:
senior_citizens = df.loc[df['Age'] > 30]
print(senior_citizens)
Scenario 2: Selecting Specific Rows and Columns
Selecting specific rows and columns simultaneously? .loc
has got you covered:
selected_data = df.loc[df['Age'] > 30, ['Name', 'City']]
print(selected_data)
Scenario 3: Modifying Data in Specific Rows and Columns
Need to modify specific data points? .loc
is perfect for targeted updates:
df.loc[df['Name'] == 'Alice', 'City'] = 'New City'
Scenario 4: Multi-level Indexing
Working with multi-level indexing? .loc
handles hierarchical data effortlessly:
selected_data = multi_index_df.loc['Group A']
print(selected_data)
Scenario 5: Conditional Data Assignment
Performing conditional assignments? .loc
makes it concise and powerful:
df.loc[df['Age'] > 35, 'Salary'] *= 2
Mastering these scenarios showcases the true potential of .loc
. From basic selections to complex conditional operations, it's your gateway to seamless data manipulation.
Scenario 6: Updating Data Based on Multiple Conditions
Apply updates to your DataFrame based on multiple conditions using .loc
. For example, increase the salary of employees older than 35 working in New York:
df.loc[(df['Age'] > 35) & (df['City'] == 'New York'), 'Salary'] += 5000
Scenario 7: Working with Multi-level Columns
Manage multi-level columns effortlessly. Access specific data points within a multi-level column structure, such as 'Sales' for 'Region A' in 2022:
df.loc[:, ('Sales', 'Region A', 2022)]
Scenario 8: Slicing Rows and Columns
Perform slicing operations to select a range of rows and columns. For example, select rows 2 to 5 and columns 'Name' to 'City':
selected_data = df.loc[2:5, 'Name':'City']
Scenario 9: Combining Advanced Indexing Techniques
Combine .loc
with other indexing techniques for complex selections. Select rows where 'Age' > 30 and columns containing the letter 'e':
selected_data = df.loc[df['Age'] > 30, df.columns[df.columns.str.contains('e')]]
Scenario 10: Assigning Data to a Subset
Assign values to a subset using .loc
. Set 'City' to 'Retired' for individuals older than 40:
df.loc[df['Age'] > 40, 'City'] = 'Retired'
With these advanced techniques, you can perform precise and complex data manipulation. .loc
is your key to handling diverse data challenges effectively.
Scenario 11: Conditional Data Update Across Multiple Columns
Apply conditional updates across multiple columns. For example, if 'Age' is greater than 35, set 'Status' to 'Senior' and double the 'Salary':
df.loc[df['Age'] > 35, ['Status', 'Salary']] = ['Senior', df['Salary'] * 2]
Scenario 12: Selecting Rows Based on String Matching
Select rows where a column contains specific text. Here, select rows where 'City' contains 'York':
selected_data = df.loc[df['City'].str.contains('York')]
Scenario 13: Selecting Rows Based on Date Ranges
Filter rows based on date ranges. For instance, select data between '2021-01-01' and '2021-12-31':
start_date = '2021-01-01'
end_date = '2021-12-31'
selected_data = df.loc[(df['Date'] >= start_date) & (df['Date'] <= end_date)]
Scenario 14: Selecting Rows Based on List of Values
Select rows where a column's value matches any in a list. For example, select rows where 'Age' is either 25 or 30:
selected_data = df.loc[df['Age'].isin([25, 30])]
Scenario 15: Handling Missing Data with .loc
Fill missing values selectively. For instance, fill missing 'Salary' values with the median 'Salary' of individuals in the same 'City':
df['Salary'].fillna(df.groupby('City')['Salary'].transform('median'), inplace=True)
Scenario 16: Conditional Data Replacement Based on Multiple Columns
Perform conditional replacements across multiple columns. For instance, if 'Age' is greater than 40 and 'Salary' is less than $50,000, set 'Status' to 'Needs Review' and increase the 'Salary' to $50,000:
df.loc[(df['Age'] > 40) & (df['Salary'] < 50000), ['Status', 'Salary']] = ['Needs Review', 50000]
Scenario 17: Advanced Multi-level Indexing and Column Selection
Utilize multi-level indexing for complex data extraction. For instance, select 'Sales' data for 'Region A' in 2022 where 'Age' is greater than 30:
selected_data = df.loc[(df['Age'] > 30) & (df['Sales', 'Region A', 2022])]
Scenario 18: Dynamic Column and Condition Selection
Dynamically select columns and apply conditions. For instance, select columns containing 'Revenue' and apply a condition where the value is greater than $100,000:
selected_columns = df.columns[df.columns.str.contains('Revenue')]
selected_data = df.loc[df[selected_columns] > 100000]
Scenario 19: Updating Data Based on External Criteria
Update data based on external criteria. For instance, if an external CSV file contains employee IDs and corresponding salary increments, update the 'Salary' column accordingly:
external_data = pd.read_csv('salary_increments.csv')
df.loc[df['EmployeeID'].isin(external_data['EmployeeID']), 'Salary'] += external_data['Increment']
Scenario 20: Complex String Matching and Extraction
Perform complex string matching and extraction using regular expressions. For example, extract all email addresses from a 'Description' column:
import re
pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b'
df['Emails'] = df['Description'].str.extractall(pattern).groupby(level=0).agg(','.join)
Mastering Advanced Data Manipulation with Pandas' .loc
Function
Scenario 21: Applying Complex Business Rules
Apply intricate business rules to your data. For example, calculate a new column 'Profitability' based on 'Revenue' and 'Expenses', but with varying rules for different product categories:
def calculate_profitability(row):
if row['Category'] == 'Electronics':
return row['Revenue'] - row['Expenses'] * 1.2
elif row['Category'] == 'Clothing':
return row['Revenue'] - row['Expenses'] * 1.5
else:
return row['Revenue'] - row['Expenses']
df['Profitability'] = df.apply(calculate_profitability, axis=1)
Scenario 22: Hierarchical Data Aggregation
Perform hierarchical data aggregation. For instance, calculate the average 'Sales' for each 'Region' and 'Year', then assign it to a new DataFrame:
aggregated_data = df.groupby(['Region', 'Year']).agg({'Sales': 'mean'}).reset_index()
Scenario 23: Handling Time Series Data
Handle time series data effectively. For example, calculate the rolling 7-day average of 'Daily Sales':
df['Rolling_Avg_Sales'] = df['Daily Sales'].rolling(window=7).mean()
Scenario 24: Advanced Grouping and Aggregation
Perform advanced grouping and aggregation. For instance, calculate the median 'Profit' for each 'Category' only for rows where 'Revenue' is above $10,000:
result = df.loc[df['Revenue'] > 10000].groupby('Category')['Profit'].median()
Scenario 25: Handling Large Datasets with Chunking
Handle large datasets efficiently using chunking. For example, process a large CSV file in chunks of 1000 rows at a time:
chunk_size = 1000
for chunk in pd.read_csv('large_dataset.csv', chunksize=chunk_size):
process_data(chunk)
Scenario 26: Merging DataFrames based on Complex Conditions
Merge DataFrames based on intricate conditions. For instance, merge 'SalesData' with 'CustomerInfo' where 'CustomerID' matches and 'PurchaseAmount' is greater than $100:
merged_data = pd.merge(SalesData.loc[SalesData['PurchaseAmount'] > 100],
CustomerInfo,
how='inner',
on='CustomerID')
Scenario 27: Recursive Data Processing with Custom Functions
Perform recursive data processing using custom functions. For example, create a function to calculate the total 'Profit' by recursively summing up the 'Profit' of all child categories:
def calculate_total_profit(category_id):
children = df.loc[df['ParentCategoryID'] == category_id]
total_profit = df.loc[df['CategoryID'] == category_id, 'Profit'].sum()
for child_id in children['CategoryID']:
total_profit += calculate_total_profit(child_id)
return total_profit
df['TotalProfit'] = df['CategoryID'].apply(calculate_total_profit)
Scenario 28: Advanced Time Series Resampling and Interpolation
Resample and interpolate time series data with advanced methods. For example, resample daily data to monthly frequency and interpolate missing values using cubic interpolation:
df.set_index('Date', inplace=True)
df_monthly = df.resample('M').mean()
df_monthly.interpolate(method='cubic', inplace=True)
Scenario 29: Real-time Data Streaming and Processing
Process real-time data streams using Pandas. For example, read and process incoming data from a WebSocket connection:
import websocket
import pandas as pd
def on_message(ws, message):
data = pd.read_json(message)
processed_data = data.loc[data['Value'] > 10]
print(processed_data)
ws = websocket.WebSocketApp("ws://data_stream_url",
on_message=on_message)
ws.run_forever()
Scenario 30: Creating Customized Statistical Models
Create customized statistical models using Pandas' versatile data manipulation capabilities. For instance, create a custom regression model that adapts to changing data patterns:
from sklearn.linear_model import LinearRegression
def adaptive_regression_model(data):
if len(data) > 1000:
model = LinearRegression()
else:
model = PolynomialRegression(degree=2)
model.fit(data[['X']], data['Y'])
return model
# Usage
model = adaptive_regression_model(df)
predicted_values = model.predict(new_data[['X']])
One more 🐼 Data Transformation with Pandas: Creating the 'joined' Column
In our journey through the world of data manipulation using Python's pandas library, we often encounter complex scenarios that demand innovative solutions. Let's explore a fascinating operation involving the DataFrame df_example
.
Consider the following line of code:
df_example.loc[:, 'joined'] = df_example.drop('key', axis=1).astype(str).apply(lambda x: ",".join(x), axis=1);
Let's break this down to understand its significance:
Breaking Down the Code:
1. df_example.drop('key', axis=1)
: This removes the 'key' column from the DataFrame df_example
.
2. .astype(str)
: Converts all remaining columns to strings.
3. .apply(lambda x: ",".join(x), axis=1)
: Concatenates the values in each row, separated by commas, creating a string.
4. df_example.loc[:, 'joined'] = ...
: Assigns the newly created comma-separated strings to a new column labeled 'joined' in df_example
.
Purpose of the Resulting 'joined' Column:
The 'joined' column consolidates data from various columns into a single, comma-separated string. This transformation enhances data organization and readability, facilitating tasks such as exporting data or performing specific analyses.
Conclusion:
The loc
function in pandas is a powerful tool for data manipulation and extraction. Its ability to select specific rows and columns using labels or boolean indexing makes it indispensable for various data analysis tasks. By mastering the usage of loc
, you can significantly enhance your data analysis capabilities in Python.
Happy coding! 🐼
Comments